Introduction
React has become one of the most popular libraries for building user interfaces, especially for single-page applications. Whether you’re working on your first React project or tackling React Homework Help, understanding the core concepts, components, state, props, and hooks will be key to completing your assignments effectively. In this detailed guide, we’ll walk you through essential React topics and provide solutions to common problems students face while learning React.
If you’re struggling with specific aspects of React or need assistance with your homework, this blog will serve as a comprehensive resource to help you navigate through it. Let’s dive into the world of React and get you started on solving your React programming challenges!
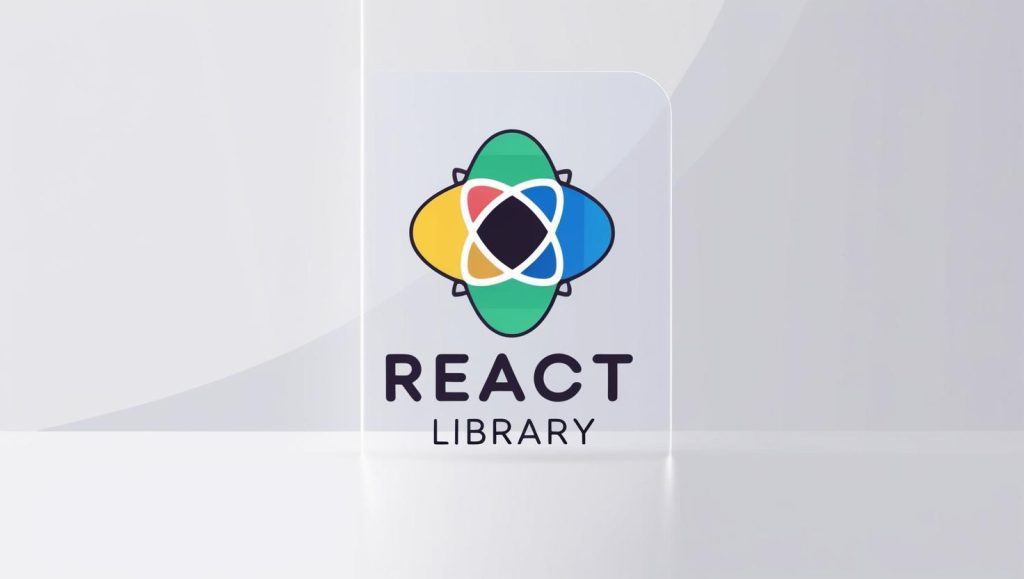
What is React?
React is a JavaScript library developed by Facebook, primarily used for building user interfaces (UI). It allows developers to create dynamic, interactive UIs with less effort by breaking down a website or app into reusable components.
Key Features of React:
- Declarative UI: React makes it easy to design interactive UIs. You describe how the UI should look based on the state of the application, and React ensures that the UI is updated when the state changes.
- Component-Based Architecture: React applications are built using components that can be reused throughout the application, making development faster and easier.
- Virtual DOM: React uses a virtual DOM to optimize rendering performance. Instead of updating the entire DOM, React only updates the parts that have changed.
For students working on React Homework Help, it’s crucial to understand the core principles of React to ensure you complete your assignments efficiently.
External Link: Learn more about React on React Official Website.
Getting Started with React
Before diving into solving specific React homework problems, you should set up your development environment. The simplest way to get started with React is to use Create React App, a tool that sets up everything you need for a React application.
- Install Node.js: React requires Node.js and npm (Node Package Manager) to manage packages. You can download it from Node.js Official Website.
- Create a New React App: Use the following command to create a new React app: bashCopyEdit
npx create-react-app my-app cd my-app npm start
This will create a basic React app and start the development server, allowing you to see your app in the browser. - Folder Structure: A typical React project structure will look like this: pgsqlCopyEdit
public/ index.html src/ App.js index.js
By getting comfortable with the React setup, you’ll be ready to solve complex problems in your React Homework Help assignments.
React Components: The Building Blocks
In React, everything is built using components. These components are JavaScript functions or classes that return UI elements.
Functional Components
A functional component is a simpler type of component written as a JavaScript function. Here’s an example:
javascriptCopyEditfunction Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
In the above example, the Welcome
component receives props
and returns a greeting. When used in another part of the app, it will render the message with the provided name
.
Class Components
While functional components are the preferred method in React (especially with the introduction of hooks), class components are still widely used. A class component is written as a JavaScript class:
javascriptCopyEditclass Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Understanding how to create and use React components is crucial for tackling React Homework Help effectively.
External Link: To learn more about components, visit React Components Documentation.
State and Props: Managing Data in React
State and props are essential concepts when working with React. They help manage data flow in your application.
Props (Properties)
Props are used to pass data from parent components to child components. They are immutable, meaning that once a prop is set, it cannot be changed by the child component. Here’s an example:
javascriptCopyEditfunction Parent() {
return <Child message="Hello from parent!" />;
}
function Child(props) {
return <h1>{props.message}</h1>;
}
In this example, the Parent
component passes a prop called message
to the Child
component.
State
State is used to store data that can change over time and affect how a component behaves. Unlike props, a component’s state can be changed using the setState
method in class components or the useState
hook in functional components. Here’s an example using useState
:
javascriptCopyEditimport React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
The Counter
component uses the useState
hook to create a count
state variable that tracks the number of button clicks. This is an essential concept when working on React Homework Help assignments.
React Hooks: A New Era of React
React introduced hooks in version 16.8 to allow functional components to use state and other React features. Before hooks, only class components could manage state, but hooks provide a cleaner and more concise way to handle state and side effects in functional components.
useState Hook
The useState
hook, as shown earlier, allows functional components to maintain state.
useEffect Hook
The useEffect
hook allows you to perform side effects in functional components, such as fetching data, updating the DOM, or subscribing to external data sources. Here’s an example:
javascriptCopyEditimport React, { useState, useEffect } from 'react';
function DataFetching() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // The empty array means the effect runs only once after the initial render
if (!data) {
return <p>Loading...</p>;
}
return <div>{data}</div>;
}
The useEffect
hook is used here to fetch data from an API when the component mounts.
Handling Forms in React
Forms are an integral part of web development, and handling form submissions in React requires understanding controlled components. A controlled component is an element whose value is controlled by React state.
javascriptCopyEditfunction MyForm() {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
alert('Name submitted: ' + name);
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
);
}
In the example above, the input field’s value is controlled by the name
state, and any changes are reflected immediately due to the onChange
event.
External Link: Explore more about forms in React on React Forms Documentation.
Conclusion
In conclusion, React Homework Help involves understanding the core principles of React, including components, props, state, hooks, and handling forms. React’s declarative nature and component-based architecture make it an excellent tool for building dynamic, interactive user interfaces. By mastering the concepts covered in this guide and practicing your React skills, you’ll be well-equipped to tackle any homework problems or assignments that come your way.
As you continue to build your React projects, be sure to consult official documentation, tutorials, and forums for help when needed. With consistent practice and understanding of the core concepts, you’ll become a proficient React developer in no time.