Introduction
Programming languages are at the core of computer science and software development. As a student working on programming languages homework, understanding the different types of programming languages and their applications is critical for success. Whether you’re struggling with assignments or simply trying to improve your coding skills, Programming Languages Homework Help can guide you through the concepts, examples, and tools you need to succeed.
In this comprehensive guide, we will explore the major programming languages used in computer science, their syntax, real-world applications, and how to approach assignments efficiently. By the end of this article, you will have the knowledge and resources needed to tackle any programming languages homework problem with confidence.
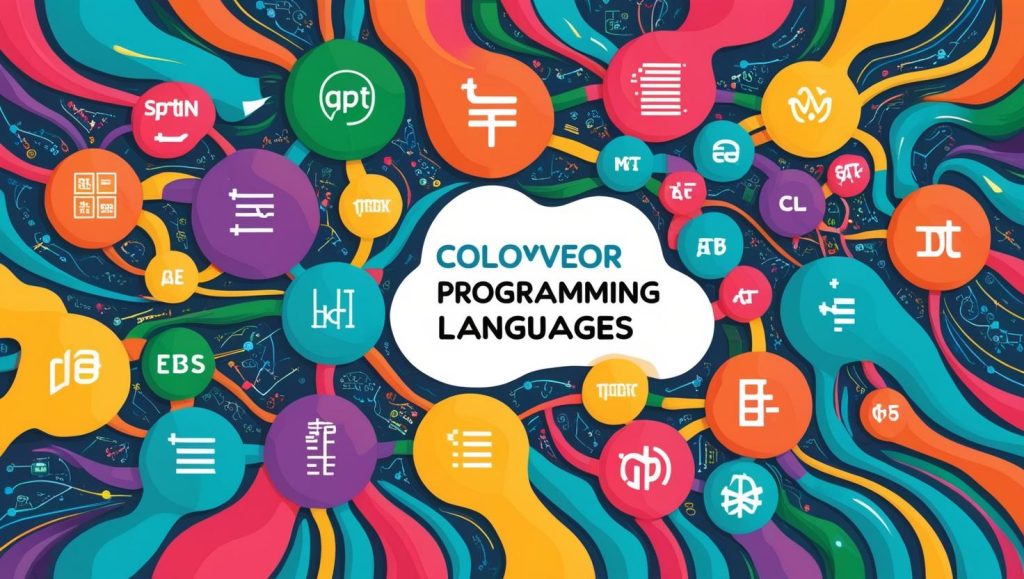
What Are Programming Languages?
Programming languages are the medium through which programmers communicate instructions to computers. These languages allow developers to create software, algorithms, and applications. Programming languages come in many varieties, each with specific strengths and weaknesses, depending on the task at hand.
Programming languages can be divided into two main categories:
- Low-Level Languages: These are closer to machine code and typically include assembly languages and machine languages.
- High-Level Languages: These are closer to human languages and are more abstract, allowing developers to write code that is easier to understand and debug.
In this section, we will discuss both low-level and high-level programming languages and provide examples for each.
External Link: For more on the history and evolution of programming languages, visit Wikipedia: Programming Languages.
Common Programming Languages for Homework Help
When it comes to Programming Languages Homework Help, it’s important to understand which languages you are most likely to encounter in your coursework. Here are some of the most popular programming languages you will come across:
1. Python
Python is one of the most versatile and beginner-friendly programming languages. It’s used in web development, data science, machine learning, artificial intelligence, and much more. Python’s syntax is clean and easy to read, which makes it perfect for students who are new to programming.
- Use Case: Data analysis, AI, web development (Django/Flask), automation.
2. Java
Java is an object-oriented programming language widely used in enterprise applications, Android app development, and large systems. Java emphasizes portability, allowing developers to write code once and run it anywhere.
- Use Case: Mobile apps (Android), enterprise software, backend development.
3. C++
C++ is an extension of the C language, adding object-oriented features. It’s commonly used in game development, systems programming, and applications that require high performance and memory management.
- Use Case: Game development, operating systems, real-time systems.
4. JavaScript
JavaScript is a client-side scripting language that’s essential for web development. It enables interactive elements on websites and is often used alongside HTML and CSS.
- Use Case: Front-end development (React, Angular), interactive web applications.
5. Ruby
Ruby is known for its simplicity and ease of use, making it a popular choice for web development. The Ruby on Rails framework helps developers build powerful applications with minimal effort.
- Use Case: Web development (Ruby on Rails), backend applications.
External Link: Explore more on programming languages at W3Schools: Programming Languages.
Understanding Syntax in Programming Languages
Each programming language has its own syntax, which dictates how the code should be written. Syntax includes things like keywords, operators, punctuation, and rules that determine the structure of the code. When working on Programming Languages Homework Help, it’s essential to understand the syntax of the language you’re using.
Syntax in Python
In Python, indentation is crucial, and the code’s structure relies on consistent indentation. Here’s a simple example of Python syntax for defining a function:
pythonCopyEditdef greet(name):
print(f"Hello, {name}!")
Syntax in Java
Java has a more rigid syntax that requires semicolons at the end of statements and curly braces for blocks of code. Here’s how you define a simple function in Java:
javaCopyEditpublic class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Data Structures in Programming Languages
One of the most important aspects of programming is understanding data structures. These are specialized formats for organizing and storing data, which help optimize the efficiency of algorithms.
1. Arrays and Lists
Arrays and lists are used to store a collection of elements. Arrays are typically used in languages like C and Java, while Python uses lists for similar purposes.
2. Stacks and Queues
Stacks and queues are used to store data in a particular order. Stacks follow the Last In First Out (LIFO) principle, while queues follow First In First Out (FIFO).
3. Trees and Graphs
Trees and graphs are more advanced data structures used to represent hierarchical and networked data, respectively. These are essential in fields like computer networking and database management.
External Link: Learn more about data structures on GeeksforGeeks: Data Structures.
Key Programming Concepts
When you’re working on Programming Languages Homework Help, you’ll encounter several key programming concepts that are critical for understanding how to write efficient and effective code. These concepts include:
1. Control Flow
Control flow refers to the order in which the instructions in a program are executed. This includes structures like if-else conditions and loops.
2. Functions and Methods
Functions allow you to encapsulate code into reusable blocks. This modular approach makes your code cleaner and easier to maintain.
3. Object-Oriented Programming (OOP)
OOP is a programming paradigm that organizes code into classes and objects. Key principles of OOP include encapsulation, inheritance, and polymorphism.
4. Recursion
Recursion occurs when a function calls itself. It’s commonly used in problems like calculating factorials or searching through tree structures.
Common Challenges in Programming Homework
Working on programming assignments can be tough, especially when you run into challenges like:
- Debugging: Debugging involves identifying and fixing errors in your code. Use tools like print statements or integrated debuggers to identify issues.
- Algorithm Design: Designing algorithms to solve problems efficiently can be difficult. Always break down the problem into smaller, manageable steps.
- Syntax Issues: Different languages have different syntax rules. A small mistake like a missing semicolon or an extra bracket can cause your code to fail.
Approaching Programming Languages Homework
When you’re assigned Programming Languages Homework Help, follow these steps for better success:
- Understand the Problem: Before jumping into coding, make sure you fully understand the problem.
- Plan the Solution: Break down the problem into smaller tasks. Create an algorithm or flowchart to outline the solution.
- Write the Code: Choose the appropriate programming language and write the code, focusing on efficiency and readability.
- Test the Code: After writing the code, test it with different input values to ensure it works as expected.
Conclusion
In conclusion, Programming Languages Homework Help can be invaluable for understanding key programming concepts, improving your coding skills, and tackling difficult assignments. By understanding different programming languages, mastering key concepts, and practicing coding techniques, you will be better prepared for success in your coursework.
If you need additional assistance, don’t hesitate to seek out online tutorials, coding communities, and tutoring services to help you succeed. Remember, programming is a skill that improves with practice, so stay persistent and keep learning!