Introduction
Programming is an essential skill for anyone pursuing a degree in computer science, software engineering, or a related field. However, many students find programming assignments to be challenging, especially when faced with tight deadlines or complex concepts. If you are looking for programming homework help, this guide is designed to assist you in understanding key programming concepts, finding solutions to common problems, and improving your coding skills.
This blog will cover fundamental programming concepts, such as algorithms, data structures, and debugging techniques. Additionally, we will highlight popular programming languages, provide tips for writing efficient code, and offer resources that will help you navigate through your programming homework with ease.
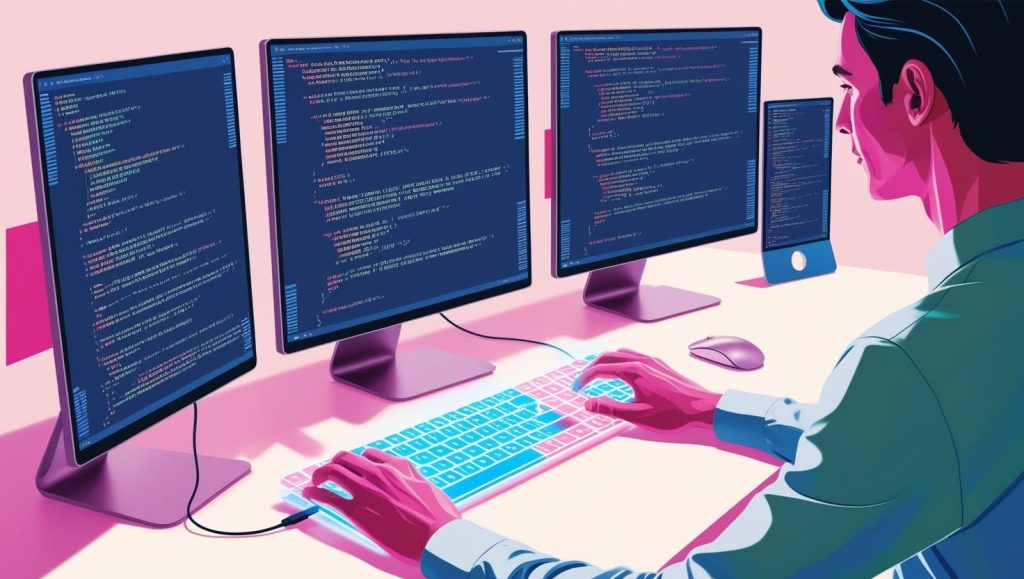
What is Programming?
Programming, also known as coding or software development, involves writing instructions that a computer can execute to perform a specific task. These instructions, known as code, are written in various programming languages like Python, Java, C++, and more.
Programming is not just about writing lines of code; it involves problem-solving, critical thinking, and understanding algorithms and data structures. It is a skill that is required in many industries, from web development to data science and artificial intelligence.
Key Concepts in Programming
If you’re struggling with programming homework help, understanding the following core concepts is essential:
- Variables and Data Types: Variables are used to store data, and data types define the type of data a variable can hold, such as integers, strings, floats, etc.
- Control Structures: These include if-else statements, for loops, and while loops, which control the flow of the program based on conditions and repetition.
- Functions and Methods: Functions allow you to organize code into reusable blocks, making your code modular and easier to manage.
- Algorithms: An algorithm is a step-by-step procedure for solving a problem. Understanding how to design efficient algorithms is crucial in programming.
External Link: For a more detailed explanation of programming concepts, visit GeeksforGeeks: Introduction to Programming.
Popular Programming Languages for Homework
One of the first decisions you will face in programming homework help is choosing the programming language to use. Here are some of the most popular languages used in programming assignments:
1. Python
Python is widely regarded as one of the easiest programming languages for beginners due to its simple and readable syntax. It is versatile, used in web development, data analysis, machine learning, and more.
2. Java
Java is known for its object-oriented principles and is used extensively in web development, Android app development, and enterprise applications.
3. C and C++
C and C++ are widely used in systems programming, game development, and applications requiring high performance.
4. JavaScript
JavaScript is primarily used for web development, enabling dynamic behavior in websites and web applications.
5. Ruby
Ruby is known for its simplicity and is often used in web development, especially with the Ruby on Rails framework.
External Link: For more on programming languages, visit W3Schools: Programming Languages.
Common Programming Homework Challenges
When you tackle programming homework help, you are likely to encounter various challenges. Below are some common obstacles and how to overcome them:
1. Debugging
Debugging is the process of identifying and fixing errors (bugs) in your code. Common debugging techniques include:
- Print Statements: Add print statements at different points in your code to trace the flow and check variable values.
- Integrated Debugger: Most IDEs (Integrated Development Environments) have built-in debuggers that let you set breakpoints and inspect variables.
External Link: Check out Stack Overflow: Debugging Techniques for additional help.
2. Understanding Algorithms
Algorithms form the backbone of many programming problems. If you’re struggling with algorithm design, it helps to break the problem down into smaller, manageable parts. Always start with a plan before you start coding.
3. Time Management
Programming assignments often come with strict deadlines. Effective time management is essential to avoid last-minute panic. Plan your tasks, break them into smaller parts, and allocate time for each task.
External Link: For tips on managing your programming time, read Harvard: Time Management for Students.
Tips for Writing Efficient Code
Writing efficient code is a crucial aspect of solving programming homework help assignments. Efficient code performs tasks with minimal resources and time. Here are a few tips:
1. Understand the Problem
Before writing any code, thoroughly read and understand the problem statement. Clarify any doubts and create a rough algorithm or flowchart to outline your solution.
2. Use Built-in Functions and Libraries
Most programming languages come with built-in functions and libraries that can save you time and effort. For example, Python’s math
library or Java’s Collections
framework.
3. Avoid Redundant Code
Ensure that your code is clean and does not have redundant lines. Use loops and functions to eliminate unnecessary repetition.
4. Optimize Time and Space Complexity
When writing algorithms, it’s crucial to focus on time and space complexity. Use Big O notation to analyze the efficiency of your code.
External Link: For more on algorithm optimization, visit GeeksforGeeks: Time Complexity.
Programming Homework Solutions
To further assist you with your programming homework help, let’s go through a sample problem:
Problem: Write a program in Python to find the factorial of a number.
Solution:
pythonCopyEditdef factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Get input from the user
num = int(input("Enter a number: "))
print("Factorial of", num, "is", factorial(num))
In this example, the function factorial()
uses recursion to find the factorial of a number. We first check if the number is 0, in which case the factorial is 1. Otherwise, we recursively call the function until we reach 0.
How to Get Programming Homework Help
If you’re stuck on a programming assignment, there are various ways to get help:
- Online Tutoring Services: Websites like Chegg and Wyzant offer one-on-one tutoring services for programming homework.
- Coding Communities: Participate in coding communities like Stack Overflow, where you can ask questions and find solutions to common programming problems.
- Programming Forums: Join forums such as Reddit’s r/learnprogramming to discuss programming problems with peers and experts.
Conclusion
In conclusion, programming homework help is essential for students looking to excel in their programming assignments and build a strong foundation in computer science. By understanding key concepts such as data structures, algorithms, and efficient coding practices, you can tackle even the most challenging homework problems. If you’re struggling with debugging or algorithm design, don’t hesitate to seek help from online resources, tutors, or programming communities.
Remember, programming is a skill that improves with practice. Keep coding, stay persistent, and seek out the resources that will help you succeed in your homework assignments.
Related Programming Homework Help Questions
Discuss the real-world applications of stacks and queues
Discuss the real-world applications of stacks and queues 2nd discussion: Discuss the real-world applications of stacks and queues beyond their use in algorithm design. How do these data structures play a critical role in systems programming, memory management, or other practical areas? Requirements: 150 – 200 words each Order Material(s) Completed File(s) Answer preview to … Read more