Introduction
Data structures and algorithms (DSA) form the backbone of computer science and programming. Whether you’re preparing for coding interviews, working on a university assignment, or just learning to code, understanding DSA is crucial for your success. However, mastering these concepts can be challenging for many students due to their complexity and abstract nature. If you’re struggling with your assignments, this comprehensive guide on Data Structures and Algorithms Homework Help will provide you with the necessary tools, strategies, and resources to excel. From basic concepts to advanced techniques, we’ll walk you through everything you need to know to complete your homework effectively.
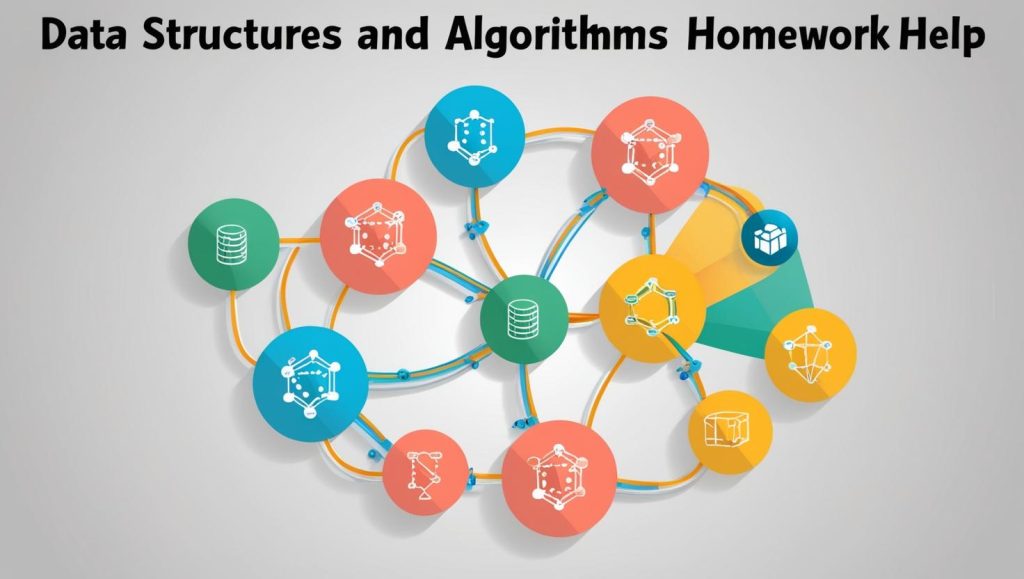
Understanding Data Structures and Algorithms: Key Concepts
Before diving into homework help, it’s important to understand what data structures and algorithms are, and why they are vital to programming.
1. What are Data Structures?
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently. The choice of data structure can significantly impact the performance of an algorithm.
Common types of data structures include:
- Arrays: A collection of elements stored in a contiguous block of memory. Elements can be accessed using an index.
- Linked Lists: A linear data structure where elements, called nodes, are stored in memory locations that are not necessarily contiguous. Each node contains data and a reference to the next node.
- Stacks: A linear data structure that follows the Last In First Out (LIFO) principle. Elements are added and removed from the top of the stack.
- Queues: A linear data structure that follows the First In First Out (FIFO) principle. Elements are added to the back and removed from the front.
- Trees: A hierarchical data structure consisting of nodes, with each node having a value and references to child nodes. Examples include binary trees, binary search trees (BST), and AVL trees.
- Graphs: A collection of nodes (vertices) and edges. Graphs can represent networks, such as social networks or transportation systems.
For a detailed breakdown of data structures, check out GeeksforGeeks—a valuable resource for learning data structures.
2. What are Algorithms?
An algorithm is a set of step-by-step instructions to solve a problem or perform a task. In programming, algorithms manipulate data structures to solve problems efficiently. The efficiency of an algorithm is often measured in terms of its time complexity and space complexity.
Some commonly used types of algorithms include:
- Sorting Algorithms: These algorithms arrange data in a specific order (e.g., quicksort, mergesort, bubble sort).
- Searching Algorithms: These algorithms are used to find a specific element in a data structure (e.g., linear search, binary search).
- Dynamic Programming Algorithms: These algorithms solve complex problems by breaking them down into simpler subproblems and using the results to solve the larger problem.
- Graph Algorithms: These algorithms are used to solve problems related to graphs (e.g., Dijkstra’s algorithm for shortest paths, breadth-first search, depth-first search).
- Greedy Algorithms: These algorithms make optimal choices at each step to achieve a globally optimal solution (e.g., Kruskal’s algorithm for minimum spanning trees).
To dive deeper into the basics of algorithms, visit TopCoder for more explanations.
How to Approach Your Data Structures and Algorithms Homework
When you encounter a Data Structures and Algorithms Homework Help assignment, the first thing you should do is approach the problem step-by-step. Here’s how you can tackle it:
1. Read and Understand the Problem
Before jumping into the coding part, make sure to fully understand the problem statement. Break it down into smaller, more manageable parts. Identify the data structure you need to use and the algorithm required to solve the problem. If necessary, sketch out the problem visually to gain a clearer understanding.
2. Choose the Right Data Structure
The first decision to make is selecting the right data structure. Ask yourself:
- What type of data am I working with (linear, hierarchical, graph-based)?
- How will the data be accessed and modified (sequentially, randomly)?
- What operations will be performed (insertions, deletions, searching, etc.)?
Choosing the right data structure is crucial for optimizing your solution and ensuring that the program runs efficiently.
For example, if your problem involves sequential data with frequent insertions and deletions, a linked list might be the best choice. On the other hand, if you’re dealing with hierarchical data, a tree would be more appropriate.
3. Write the Algorithm
Once you’ve identified the correct data structure, write the algorithm. Focus on writing clean, modular code that clearly solves the problem. Break the solution into smaller, logical steps and write helper functions if necessary. Make sure to handle edge cases.
4. Optimize the Code
After you have written the basic solution, analyze its time complexity and space complexity. Aim for the most efficient algorithm possible in terms of these two factors. Use Big-O notation to evaluate your algorithm’s performance and make improvements if needed.
For additional guidance on algorithm optimization, visit Big-O Cheat Sheet for a comprehensive reference.
5. Test Your Solution
Once your solution is implemented, test it with multiple test cases to ensure it works as expected. Check for corner cases and edge cases to make sure your solution is robust and can handle unexpected inputs.
Common Data Structures and Algorithms Problems
Here are some common problems that students encounter while solving DSA homework, along with helpful strategies for solving them:
1. Reversing a Linked List
The goal of this problem is to reverse a singly linked list. To solve it, you’ll need to iterate through the list, changing the direction of the pointers at each node.
2. Binary Search
Binary search is an efficient algorithm used to search for an element in a sorted array. The idea is to repeatedly divide the search interval in half and eliminate half of the remaining elements.
For more about binary search, check out Binary Search Explained.
3. Finding the Shortest Path in a Graph
In problems involving graphs, you might be asked to find the shortest path between two nodes. Algorithms like Dijkstra’s Algorithm or Bellman-Ford Algorithm can be used to solve these problems.
Learn about Dijkstra’s algorithm from this Tutorial.
4. Sorting Algorithms
Sorting is a fundamental problem in computer science. You may encounter problems that require you to implement sorting algorithms like quicksort, mergesort, or insertion sort.
Explore more about sorting algorithms on GeeksforGeeks Sorting Algorithms.
External Resources for Data Structures and Algorithms Homework Help
If you’re struggling with your homework, here are some valuable external resources that can assist you:
- GeeksforGeeks
A comprehensive online resource for learning data structures, algorithms, and programming concepts.
Link: https://www.geeksforgeeks.org/ - LeetCode
An excellent platform for practicing coding problems and preparing for technical interviews.
Link: https://leetcode.com/ - HackerRank
Another great platform for practicing coding challenges and improving problem-solving skills.
Link: https://www.hackerrank.com/domains/tutorials/10-days-of-javascript - Visualgo
A visual tool to help you understand and visualize data structures and algorithms.
Link: https://visualgo.net/en - Data Structures and Algorithms by Robert Lafore
A comprehensive textbook that covers both theoretical and practical aspects of data structures and algorithms.
Link: Amazon Link
Tips for Excelling in Data Structures and Algorithms Homework
Here are some tips that can help you tackle your DSA homework effectively:
1. Practice Regularly
Data structures and algorithms require consistent practice. Solve problems on platforms like LeetCode, HackerRank, or Codeforces to improve your problem-solving skills.
2. Understand the Time and Space Complexity
Always analyze the time and space complexity of your solutions. Aim to write the most efficient code possible.
3. Work on Projects
Working on real-world projects that involve DSA concepts will help reinforce your learning and make the theory come alive.
4. Seek Help When Stuck
If you’re stuck on a problem, don’t hesitate to ask for help. Discuss your solutions with classmates, consult online forums like StackOverflow, or refer to detailed tutorials.
Conclusion
In conclusion, Data Structures and Algorithms Homework Help is crucial for your success in computer science. Understanding and applying DSA concepts can seem daunting at first, but with the right approach and consistent practice, you can master these topics. Whether you’re working on linked lists, sorting algorithms, or graph problems, this guide has provided you with the necessary tools to approach your homework with confidence. Be sure to practice regularly, use external resources, and optimize your solutions for efficiency.