Introduction
C# (C-Sharp) is a popular, versatile programming language developed by Microsoft. It is widely used for developing desktop applications, web applications, game development, and enterprise solutions. Many students seek C# homework help to understand object-oriented programming, syntax, data structures, and .NET framework integration. This guide provides a detailed overview of C#, key concepts, applications, challenges, and resources for students to master the language.
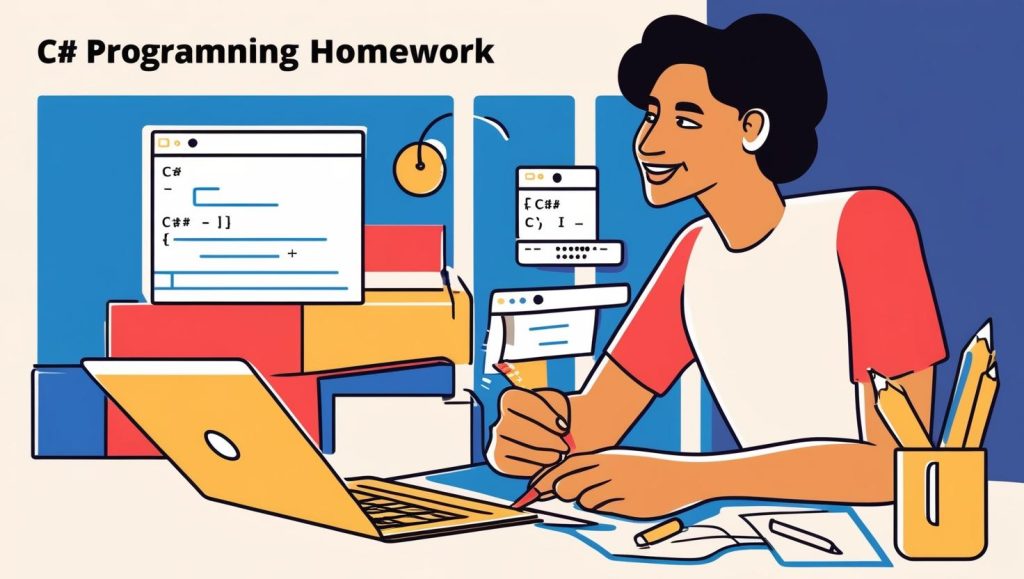
What is C#?
C# is a modern, object-oriented programming language that is part of the .NET framework. It is known for its simplicity, strong type-checking, and automatic memory management through garbage collection. C# is commonly used in:
- Windows Application Development – Using Windows Forms and WPF.
- Web Development – Through ASP.NET and Blazor.
- Game Development – With Unity.
- Enterprise Applications – For business software solutions.
To learn more about C#, visit the Microsoft C# Documentation.
Key Concepts in C#
1. Object-Oriented Programming (OOP)
C# is an object-oriented language, meaning it follows OOP principles:
- Encapsulation – Protecting data through access modifiers.
- Inheritance – Allowing code reuse between classes.
- Polymorphism – Enabling methods to operate on different data types.
- Abstraction – Hiding implementation details from the user.
2. C# Syntax and Data Types
C# provides built-in data types, including:
- int – Integer numbers.
- double – Floating-point numbers.
- string – Text data.
- bool – Boolean values (true/false).
- char – Single character.
3. Exception Handling
C# uses try
, catch
, and finally
blocks for handling errors gracefully. Example:
try {
int num = Convert.ToInt32("abc"); // This will cause an exception
} catch (Exception ex) {
Console.WriteLine("Error: " + ex.Message);
} finally {
Console.WriteLine("Execution completed.");
}
For a deeper dive, check C# Exception Handling Guide.
4. LINQ (Language Integrated Query)
LINQ allows querying collections in a SQL-like manner. Example:
var numbers = new List<int> {1, 2, 3, 4, 5};
var evenNumbers = numbers.Where(n => n % 2 == 0);
Learn more at LINQ Tutorial.
5. .NET Framework and Core
C# runs on the .NET platform, which includes:
- .NET Framework – Traditional Windows-based development.
- .NET Core – Cross-platform development for Windows, macOS, and Linux.
- ASP.NET – For building web applications.
Applications of C#
C# is widely used in various domains:
- Desktop Applications – Microsoft Office plugins, Windows Forms, WPF apps.
- Web Development – ASP.NET MVC and Blazor web applications.
- Game Development – Unity-based 2D and 3D games.
- Mobile Development – Xamarin for cross-platform mobile apps.
- Machine Learning – ML.NET for AI-powered applications.
For real-world applications, visit Microsoft Learn.
Challenges in Learning C#
Students often struggle with:
- Understanding OOP concepts.
- Debugging runtime errors.
- Mastering LINQ queries.
- Working with asynchronous programming (async/await).
Seeking C# homework help can make learning these concepts easier.
How to Get C# Homework Help
1. Online Courses
Learn C# from platforms like:
2. C# Books
Recommended books include:
- “C# in Depth” by Jon Skeet.
- “Head First C#” by Andrew Stellman.
- “Pro C# 9 with .NET” by Andrew Troelsen.
3. C# Coding Practice
Improve your coding skills at:
4. Discussion Forums
Engage with the C# community:
Best Practices for Studying C#
- Understand OOP principles before diving into complex applications.
- Write clean, readable code following naming conventions.
- Practice with real-world projects to gain hands-on experience.
- Use debugging tools like Visual Studio’s Debugger.
- Explore open-source C# projects on GitHub.
By following these best practices, students can master C# efficiently.
Conclusion
C# is a powerful language used in multiple domains, from web development to game programming. Students looking for C# homework help can take advantage of online courses, books, coding platforms, and community forums to enhance their skills. Mastering C# requires dedication, hands-on practice, and the right learning resources.